C program Simple Interest and Compound Interest
This is a C programming code which calculates banking interest on principle amount for N years using Simple interest formula and Compound Interest formula. The input values for principle amount and number of years is read from user.
Simple interest - formula
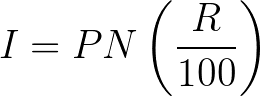
- I - Interest Amount
- P - Principle Amount to be deposited
- N - Number of years the principle amount to be deposited
- R - Rate of interest per year in percentage
Simple interest - example calculation
Assume that, we are going to deposit 5000$ in bank at rate of interest 8% for 5 years, calculate simple interest for the principle amount after 5 years P=5000; R =8; N=5; I =? I = 5000 x 5 x 8/100 I = 25000 x 0.08 I = 2000$ after 5 years , we will get additionally 2000$ as interest along with principle amount (10000$).
Simple Interest - C programming code
#include <stdio.h>
#include<math.h>
int main() {
float A,R,N;
float si=0;
printf ("Find a simple interest for principle amount");
printf("\n Enter principle amount :" );
scanf("%f", &A);
printf("\n Enter interest in percentage :" );
scanf("%f", &R);
printf("\n Enter number of year :" );
scanf("%f", &N);
si = A * (R/100) * N;
printf("Simple Interest is : %.2f",si);
printf(" Total Amount after %.1f is : %.2f",(A+si));
return 0;
}
Simple Interest - C programming output
Find a simple interest for principle amount Enter principle amount :5000 Enter interest in percentage :8 Enter number of year :5 Simple Interest after 5.0 is : 2000.00
Compound interest - formula
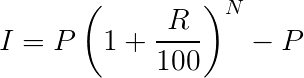
- I - Interest Amount
- P - Principle Amount to be deposited in bank
- N - Number of years the principle amount to be deposited
- R - Rate of interest per year in percentage
Compound interest - example calculation
In compound interest - The principle amount is increased every year due to accumulating interest of principle amount with principle amount. so that,finally compound interest gives greater value than simple interest.
Assume that, we are going to deposit 5000$ in bank at rate of interest 8% for 5 years, calculate compound interest for the principle amount after 5 years P=5000; R =8; N=5; I =? I = 5000 x (1 + 8/100)5 - 5000 I = 5000 x (1 + 0.08)5 - 5000 I = 5000 x (1.08)5 - 5000 I = 5000 x 1.46932 - 5000 I = 7346 - 5000 I = 2346$ after 5 years , we will get additionally 2346$ as interest along with principle amount (10000$).
Compound Interest - C programming code
#include <stdio.h>
#include<math.h>
int main() {
float A;
float R;
float N;
float ca=0;
printf ("Find a compound interest for principle amount");
printf("\n Enter principle amount :" );
scanf("%f", &A);
printf("\n Enter interest in percentage :" );
scanf("%f", &R);
printf("\n Enter number of year :" );
scanf("%f", &N);
ca = A * pow( (1+R/100),N);
printf(" Compound Interest after %.1f is : %.2f",(ca-A));
printf(" Total Amount plus principle amount after %.1f is : %.2f",ca);
return 0;
}
Compound Interest - C program Output
Find a compound interest for principle amount Enter principle amount :5000 Enter interest in percentage :8 Enter number of year :5 Compound Interest after 5.0 is : 2346.64 Total Amount plus principle amount after 5.0 : 7346.64
Comments
Post a Comment