C program Simple Interest and Compound Interest
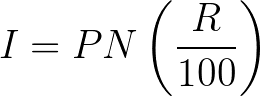
This is a C programming code which calculates banking interest on principle amount for N years using Simple interest formula and Compound Interest formula. The input values for principle amount and number of years is read from user. Simple interest - formula I - Interest Amount P - Principle Amount to be deposited N - Number of years the principle amount to be deposited R - Rate of interest per year in percentage Simple interest - example calculation Assume that, we are going to deposit 5000$ in bank at rate of interest 8% for 5 years, calculate simple interest for the principle amount after 5 years P=5000; R =8; N=5; I =? I = 5000 x 5 x 8/100 I = 25000 x 0.08 I = 2000$ after 5 years , we will get additionally 2000$ as interest along with principle amount (10000$)